❮ 2023-11-29
Glacier CTF 2023 Writeups
CTF Writeups for GlacierCTF
Jeopardy CTF with local group from Braunschweig, were not that active active for that CTF though, I was only able to solve beginner challenges.
Glacier Exchange (Web)
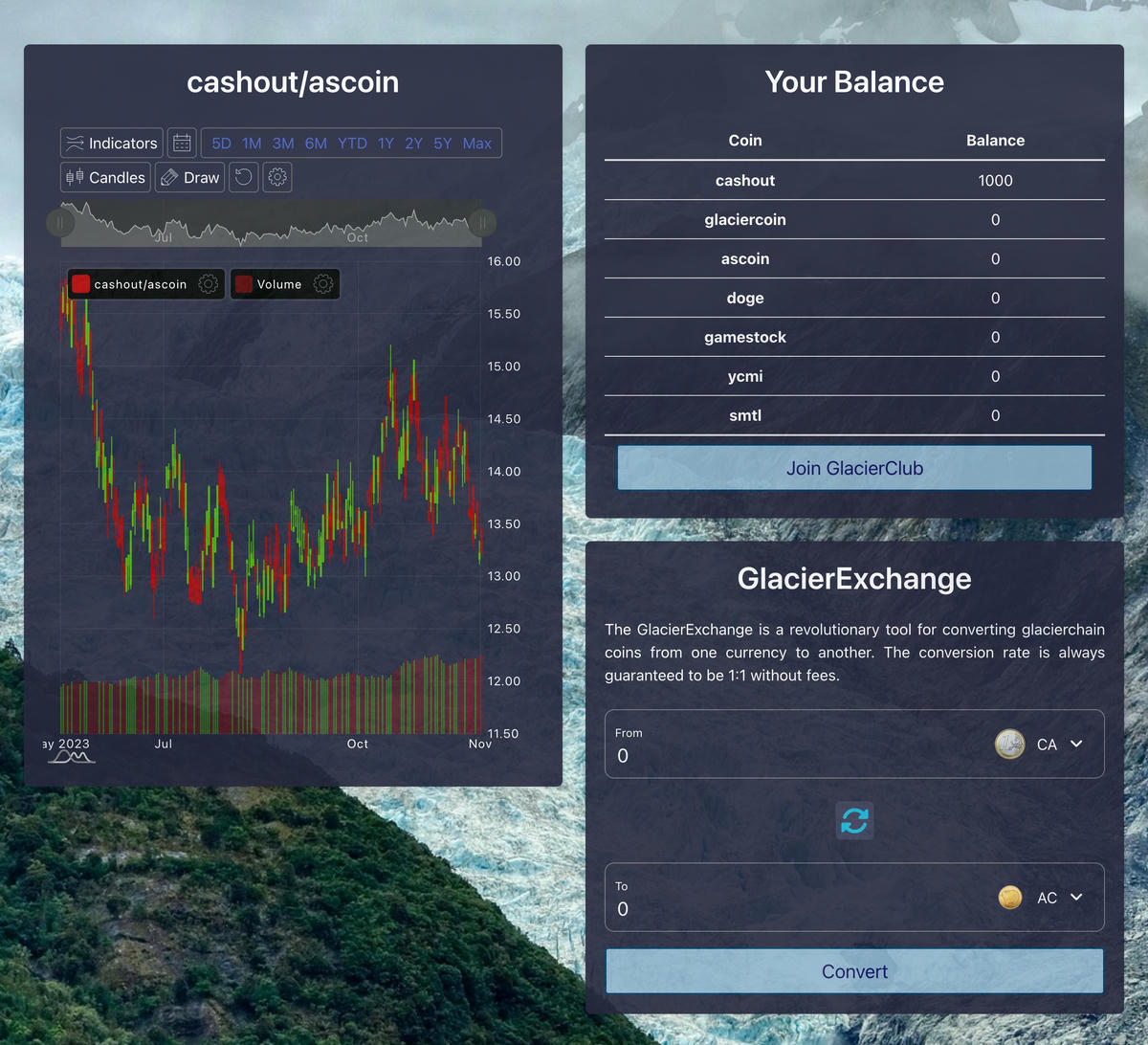
Goal of this task was to get 1 000 000 000 Dollars in Cash-Out while having nothing in your other wallets. Source Code was provided. A quick look at the source code revealed, that you could trade a negative sums of money, so if you "invest" -999 Dollar of your cash-out money to something else you get more money in your cash-out account.
-=
+=
return 1
return 0
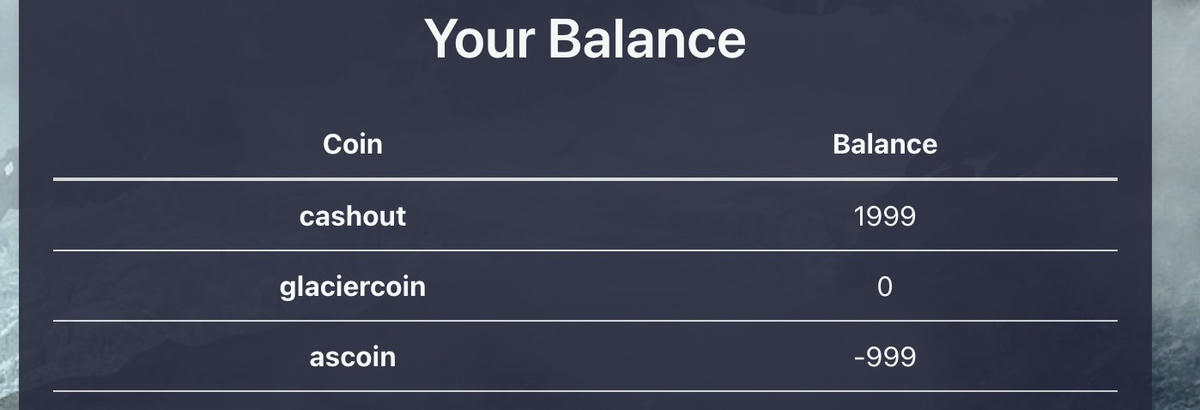
So just trade minus one-billion dollars and get the flag? Not so fast, you still have to zero out your "dept" of your other wallets first, but how?
Internally, the backend is a python flask application, and the wallet balance is stored as float, in python these numbers cannot overflow, but if the numbers stored within are getting too large the float switches to "Inf".
Transferring "sys.float_info.max" amount of money (1.7976931348623157e+308) from your cash-out wallet to another wallet twice gets you plus Infinity Money. Congratz!
Now your can "substract" any amount of Inifinity from your endless wealth to zero out the other wallets, to prevent these from reaching minus Infinity, just spread both transactions to different wallets.
Once you zero out both wallets you get the flag once you enter the "club"
Exploit:
#cookie="eyJpZCI6IjJhY0tKQmJZSzZreW1pWTdYNjBMaXcifQ.ZWG62A.-aiJuSTRvDHgo0Kgo6NR7xOBg5A"
=
=
=
=
=
=
=
Output:
}
} } } } } } }
}
} } } } } } }
}
} } } } } } }
}
} } } } } } }
} } } } } } }
}
Skilift (Beginner Misc)
This challenge was quite straightforward, you needed to provide a code and you had the source on how the backend converts your input before checking it. Only hard part: The Code was written in Verilog.
1 2 3 4 ;
5
6 reg tmp1, tmp2, tmp3, tmp4;
7
8 // Stage 1
9 always begin
10 tmp1 = key & 64'hF0F0F0F0F0F0F0F0;
11 end
12
13 // Stage 2
14 always begin
15 tmp2 = tmp1 <<< 5;
16 end
17
18 // Stage 3
19 always begin
20 tmp3 = tmp2 ^ "HACKERS!";
21 end
22
23 // Stage 4
24 always begin
25 tmp4 = tmp3 - 12345678;
26 end
27
28 // I have the feeling "lock" should be 1'b1
29 assign lock = tmp4 == 64'h5443474D489DFDD3;
30
31 endmodule
"Strategy" on how to solve it: Just reverse any action the code does, only the first Stage is hard, because a AND Operation is not reversible, maybe brute-force this step?
This code, that just ignored the AND stage worked on the first try, guess the challenge was designed in a way that the AND Input was just the AND Output:
= 0xF0F0F0F0F0F0F0F0
=
= 0x5443474D489DFDD3
= 12345678
= 64
= +
=
= ^
= >> 5
=
Challenge:
)
>0
}
Solves from others I found interesting
Silent Snake (Misc)
Challenge: You are able to fire one "ls" to show directory contents, everything else is forbidden and won't work.
1 #!/usr/bin/env python3
2
3
4
5
6
7 = ==
8
9 =
10 =
11
12 13 14 15 16 17 18 19 20 21
22
23
24
25
26
27
28 =
29 =
30
31
32
33
34
35
36 # Redirect stderr to stdout
37
38
39
40
41
42
43
44
I wrote some code to scan every folder recursive inside the system, but that did not lead to finding a flag. After the competition ended I looked at the misc discord channel and someone posted their solution.
What I did not think of: "run_command(ls /)" was not the only command I could run, because I had an (invisible) interactive shell I could have chained arbitrary code before or after the run_command command and do stuff there.
The smart person from the discord server opened the flag and brute forced every character, if the "contains" function found a matching character in the flag.txt file only then he would run the "run_command(ls / )" function.
Every time the script would return the content of ls another new character of the flag have been found.
The Code was:
#!/bin/bash
flag="gctf{"
found="true"
while ; do
found="false"
for ; do
if ; then
continue
fi
| | && found="true" && flag+=
done
done
The Code in action:
My first Website (Beginner Web)
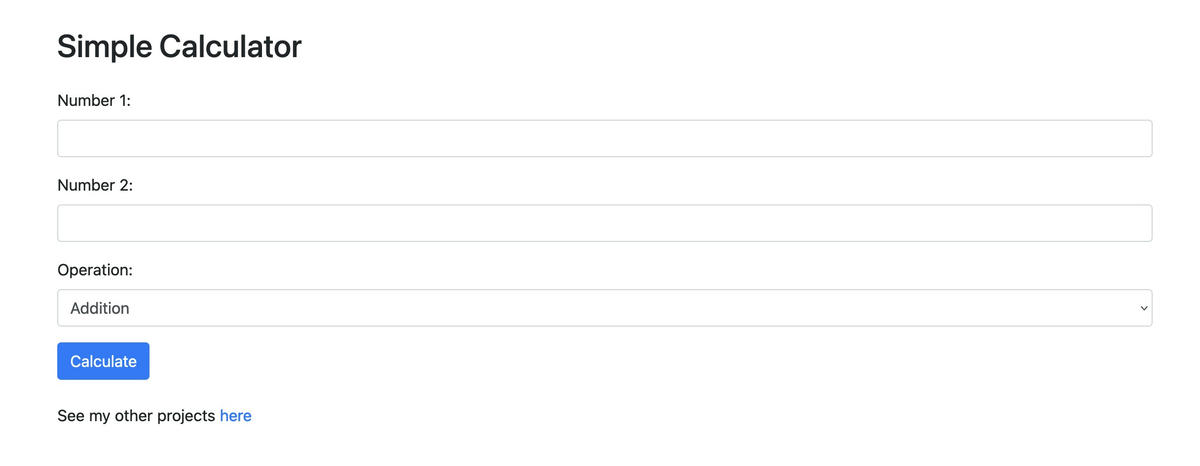
The /project hyperlink lead to a 404 page, the http response itself was 404 though. The calculator stuff was a hint that a server side templating-engine was used. I also did not think of this
The only controllable stuff for me was the url path. Turns out the "path" was also used inside the templating engine, it printed "xy was not found on the server", so we can inject template code via the url path parameter:

Flag:
